Installation
This document will guide you through the process of adding AppSpector SDK to your project and tailoring it to your needs. Its simplified but sufficient for a quick setup version can be found in "Setup Guide" section on your project page on our website.
Each app you want to use with AppSpector SDK you have to register on the web (https://app.appspector.com).
After adding the application navigate to app settings and copy API key.
To use AppSpector on tvOS just follow installation steps below but use AppSpectorTVSDK instead.
You can add AppSpector SDK to your project manually or via CocoaPods/Carthage/SPM
Manually
To manually link just download AppSpectorSDK.framework and drop AppSpectorSDK.framework to your XCode project.
Then navigate to your project settings and under 'General' tab add AppSpectorSDK framework to 'Embedded Binaries' section.
If you plan either to submit builds with AppSpector SDK to the Apple TestFlight for testing or archive them for AdHoc distribution you'll have to perform one more step: create a new “Run Script Phase” in your app’s target’s “Build Phases” and paste the following script:
code_sign() {
echo "Code Signing $1 with Identity ${EXPANDED_CODE_SIGN_IDENTITY_NAME}"
echo "/usr/bin/codesign --force --sign ${EXPANDED_CODE_SIGN_IDENTITY} --preserve-metadata=identifier,entitlements $1"
/usr/bin/codesign --force --sign ${EXPANDED_CODE_SIGN_IDENTITY} --preserve-metadata=identifier,entitlements "$1"
}
cd "${BUILT_PRODUCTS_DIR}/${FRAMEWORKS_FOLDER_PATH}"
for file in $(find . -type f -perm +111); do
if ! [[ "$(file "$file")" == *"dynamically linked shared library"* ]]; then
continue
fi
archs="$(lipo -info "${file}" | rev | cut -d ':' -f1 | rev)"
stripped=""
for arch in $archs; do
if ! [[ "${VALID_ARCHS}" == *"$arch"* ]]; then
lipo -remove "$arch" -output "$file" "$file" || exit 1
stripped="$stripped $arch"
fi
done
if [[ "$stripped" != "" ]]; then
echo "Stripped $file of architectures:$stripped"
if [ "${CODE_SIGNING_REQUIRED}" == "YES" ]; then
code_sign "${file}"
fi
fi
done
This script is required as a workaround for this Apple AppStore bug
CocoaPods
To use cocoapods, add this line to your podfile and run pod install
:
pod 'AppSpectorSDK'
To get SDK version with encryption feature use AppSpectorE2E
pod:
pod 'AppSpectorSDKE2E'
Carthage
- Install Carthage
- Add
binary "https://github.com/appspector/ios-sdk/raw/1.4.0/AppSpector.json"
to your Cartfile - Run
carthage update
- Drag AppSpectorSDK.framework from the appropriate platform directory in Carthage/Build/ to the “Linked Frameworks and Libraries” section of your Xcode project’s “General” settings
To get SDK version with encryption feature use AppSpectorE2E
link:
https://raw.githubusercontent.com/appspector/ios-sdk/1.4.0/AppSpectorE2E.json
SPM
AppSpector supports SPM but unfortunately not for E2E version.
Long story short: we have to wait for guys from openssl to support Apple Sillicon,
so if you need version with end-to-end encryption please refer to the main repo (https://github.com/appspector/ios-sdk) and use one of the installation methods available.
For the rest of you: to install SDK via SPM just press '+' sign in Xcode list of packages and paste repo address: 'https://github.com/appspector/ios-sdk-spm' into the search field:
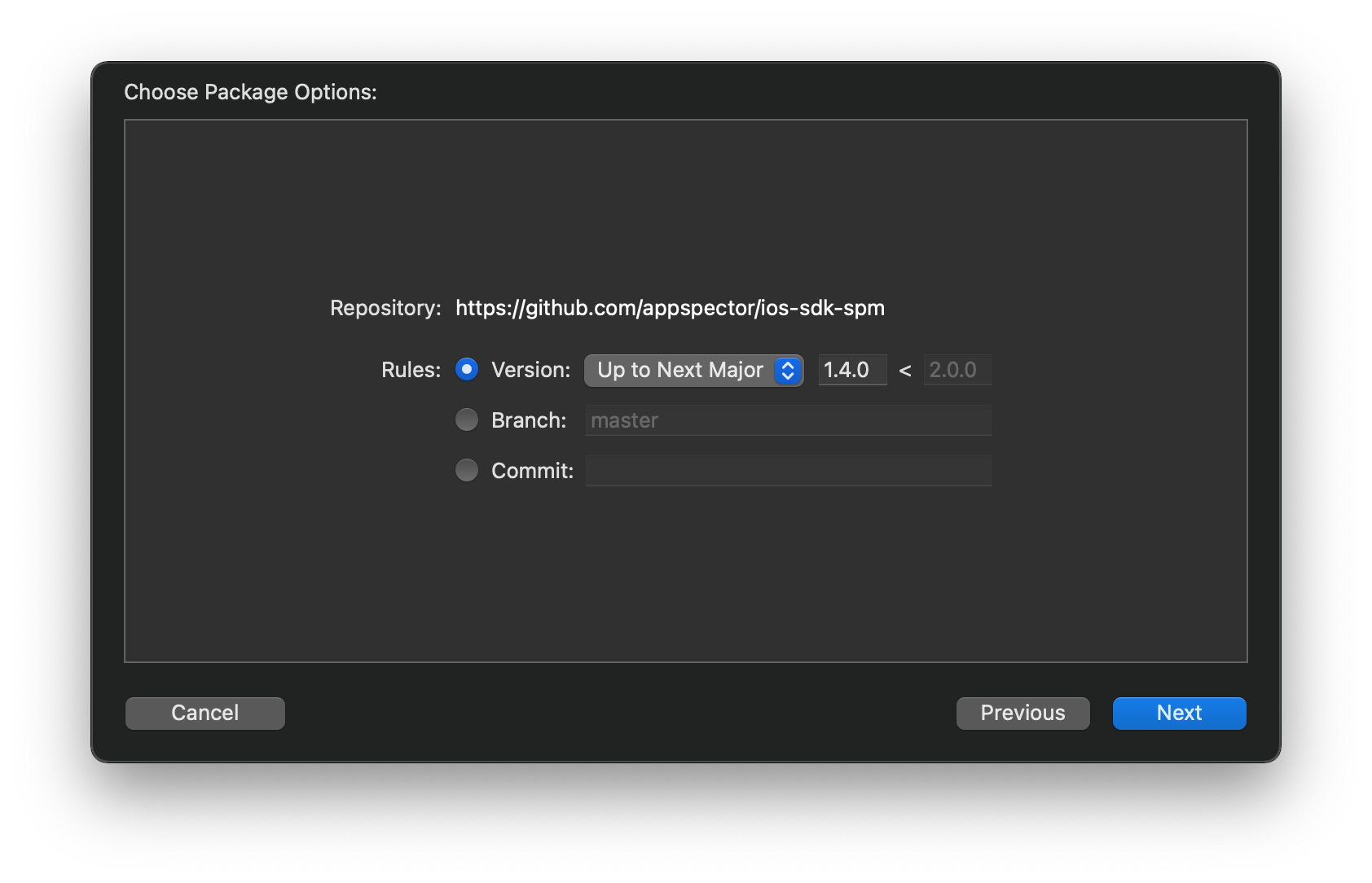
Two targets will be available: for iOS and tvOS, choose which one you need, press 'next' and you are done:
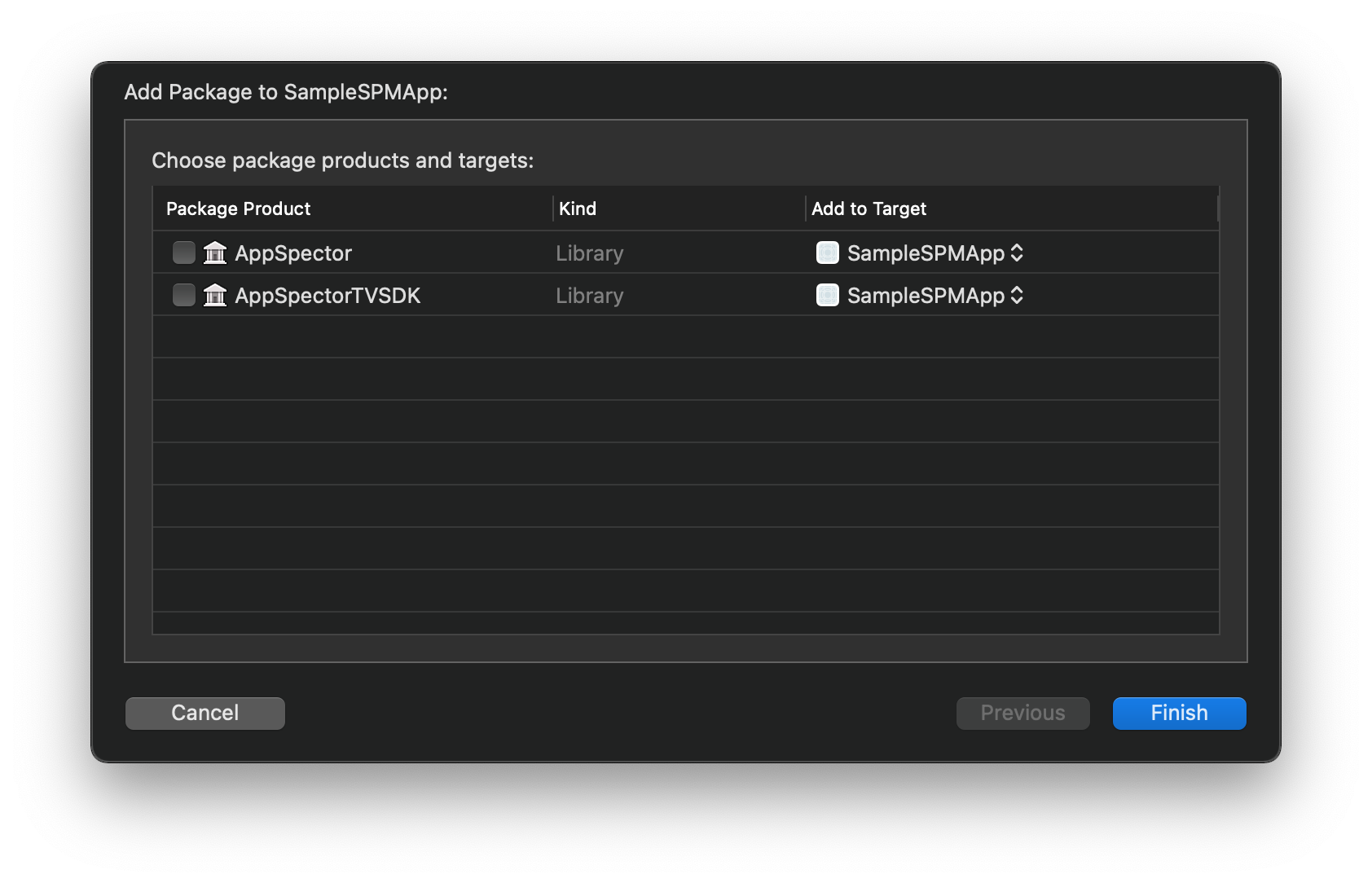
SwiftUI integration
As of SwiftUI 2 Apple completely switched apps to a new lifecycle where there is no AppDelegate by default. Developers doesn't have handy didFinishLaunchingWithOptions()
method anymore but there are still some points where you can put AppSpector SDK initialization code:
App's init()
init()
You can add init()
to your apps struct and put SDK init code there:
@main
struct MyApp: App {
init() {
let config = AppSpectorConfig(apiKey: "API_KEY")
AppSpector.run(with: config)
}
var body: some Scene {
WindowGroup {
ContentView()
}
}
App Delegate
You can use App Delegate class with old app lifecycle functions everyone used to:
class AppDelegate: NSObject, UIApplicationDelegate {
func application(_ application: UIApplication,
didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey : Any]? = nil) -> Bool {
let config = AppSpectorConfig(apiKey: "API_KEY")
AppSpector.run(with: config)
return true
}
}
@main
struct MyApp: App {
@UIApplicationDelegateAdaptor(AppDelegate.self) var delegate
var body: some Scene {
WindowGroup {
ContentView()
}
}
}
Apple TV
AppSpector is also available for tvOS, you can use any of described above methods to install it, all you need is just use AppSpectorTVSDK
pod instead of AppSpectorSDK
and include AppSpectorTVSDK.framework
instead of AppSpectorSDK.framework
.
Updated almost 3 years ago